Windows Recent File List
Windows Recent File List
This is going to be a strange one. Hopefully I can describe it properly.
If I do a "Save as PDF" on a drawing, and then go to outlook, that pdf appears in my "Recent Items" list (for example, if I want to attach that file to an email.)
However, if I run the macro I created that does the same "Save as PDF" process, it does NOT appear in my "Recent Items" list. Do any of you API guru's know if I can add something to my macro to change this behavior?
If I do a "Save as PDF" on a drawing, and then go to outlook, that pdf appears in my "Recent Items" list (for example, if I want to attach that file to an email.)
However, if I run the macro I created that does the same "Save as PDF" process, it does NOT appear in my "Recent Items" list. Do any of you API guru's know if I can add something to my macro to change this behavior?
-
I may not have gone where I intended to go, but I think I have ended up where I needed to be. -Douglas Adams
I may not have gone where I intended to go, but I think I have ended up where I needed to be. -Douglas Adams
Re: Windows Recent File List
Add this enum to your namespace:
Code: Select all
public enum ShellAddToRecentDocsFlags
{
Pidl = 0x001,
Path = 0x002,
PathW = 0x003
}
Code: Select all
[DllImport("Shell32.dll", BestFitMapping=false, ThrowOnUnmappableChar=true)]
private static extern void SHAddToRecentDocs(ShellAddToRecentDocsFlags flag, [MarshalAs(UnmanagedType.LPStr)]string path);
Code: Select all
mExt.SaveAs(path, (int)swSaveAsVersion_e.swSaveAsCurrentVersion, (int)swSaveAsOptions_e.swSaveAsOptions_Silent, exportData, ref errors, ref warnings);
SHAddToRecentDocs(ShellAddToRecentDocsFlags.Path, path);
Re: Windows Recent File List
This is exactly what I was looking for. Thank you!
-
I may not have gone where I intended to go, but I think I have ended up where I needed to be. -Douglas Adams
I may not have gone where I intended to go, but I think I have ended up where I needed to be. -Douglas Adams
- CarrieIves
- Posts: 133
- Joined: Fri Mar 19, 2021 11:19 am
- Location: Richardson, TX
- x 313
- x 112
Re: Windows Recent File List
@JSculley ,
My save as PDF macro is in VBA. Are the code snippets you provided in VBA or something else? I'm still learning my way around macros for SolidWorks.
Thanks,
Carrie
My save as PDF macro is in VBA. Are the code snippets you provided in VBA or something else? I'm still learning my way around macros for SolidWorks.
Thanks,
Carrie
Re: Windows Recent File List
The snippets are C#. You can do the same sort of thing with VBA. Add these constants:CarrieIves wrote: ↑Tue Jan 25, 2022 11:20 am My save as PDF macro is in VBA. Are the code snippets you provided in VBA or something else? I'm still learning my way around macros for SolidWorks.
Code: Select all
Const Pidl = 1
Const Path = 2
Const PathW = 3
Code: Select all
Private Declare PtrSafe Sub SHAddToRecentDocs Lib "shell32" _
(ByVal uFlags As Long, ByVal pv As Any)
Code: Select all
SHAddToRecentDocs Path, filePath
- CarrieIves
- Posts: 133
- Joined: Fri Mar 19, 2021 11:19 am
- Location: Richardson, TX
- x 313
- x 112
Re: Windows Recent File List
@JSculley THANKS 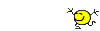
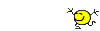
Re: Windows Recent File List
I tested it this morning and it works perfectly. Thanks again @JSculley
-
I may not have gone where I intended to go, but I think I have ended up where I needed to be. -Douglas Adams
I may not have gone where I intended to go, but I think I have ended up where I needed to be. -Douglas Adams
- Jaylin Hochstetler
- Posts: 383
- Joined: Sat Mar 13, 2021 8:47 pm
- Location: Michigan
- x 375
- x 353
- Contact:
Re: Windows Recent File List
FYI,
If you ever want to do this in .NET, which I did recently your code needs to look like this
Granted, I'm sure there are other ways of doing it too.
If you ever want to do this in .NET, which I did recently your code needs to look like this
Code: Select all
Public Enum ShellAddToRecentDocsFlags
Pidl = &H1
Path = &H2
PathW = &H3
End Enum
<DllImport("Shell32.dll", BestFitMapping:=False, ThrowOnUnmappableChar:=True)>
Private Shared Sub SHAddToRecentDocs(ByVal flag As ShellAddToRecentDocsFlags,
<MarshalAs(UnmanagedType.LPStr)> ByVal path As String)
A goal is only a wish until backed by a plan.